How to create a responsive sidebar using HTML, CSS and JavaScript.
Posted on 2024-09-18
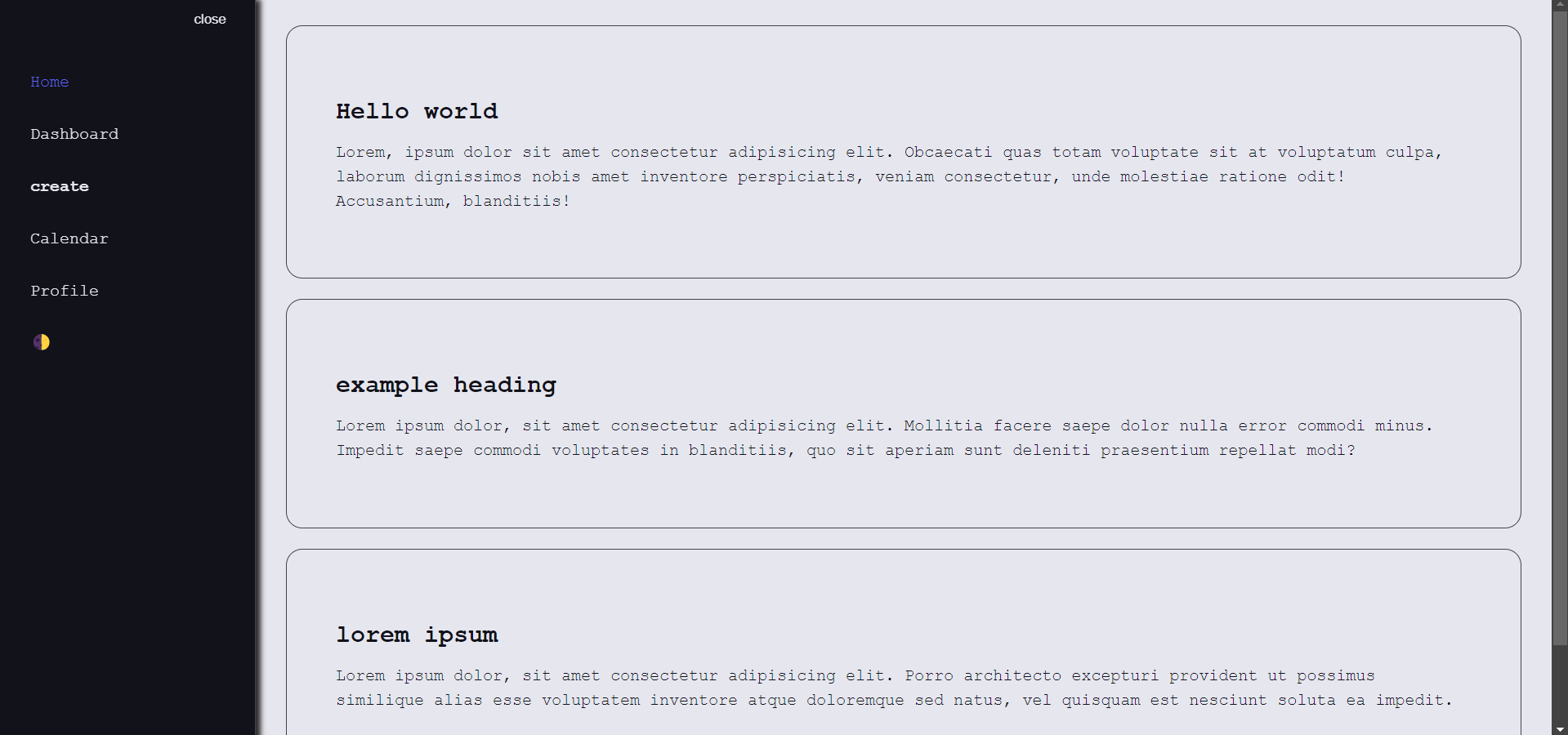
Hello, everyone! ๐ Today, we are going to learn how to create a responsive sidebar using HTML, CSS, and JavaScript. What will you gain from following this tutorial? I hope you will enhance your CSS styling skills and improve your JavaScript logic.
read also How to create a simpe calculator using HTML CSS and JavaScript
Firstly, we need to create a folder. You can name it "responsive-sidebar" or anything you prefer. Then, we will create HTML, CSS and JavaScript files within that folder. Name the HTML file "index.html", CSS file "style.css" and the JavaScript file "app.js".
In the HTML file, we will set the title within the 'head' tags. Name the title "sidebar". In the 'body' element, we add 'ul' and 'li' list element within 'nav' element.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>sidebar</title>
<link rel="stylesheet" href="style.css">
<script type="text/javascript" src="app.js" defer></script>
</head>
<body>
<nav id="sidebar">
<ul>
<li>
<button id="toggle-btn" onclick=toggleSideBar() >close</button>
</li>
<li class="active">
<a href="index.html">
<span>Home</span>
</a>
</li>
<li>
<a href="dashboard.html">
<span>Dashboard</span>
</a>
</li>
<li>
<button onclick=toggleSubMenu(this) class="dropdown-btn">
<span>create</span>
</button>
<ul class="sub-menu">
<div>
<li>
<a href="">folder</a>
</li>
<li>
<a href="">document</a>
</li>
<li>
<a href="">project</a>
</li>
</div>
</ul>
</li>
<li>
<a href="calendar.html">
<span>Calendar</span>
</a>
</li>
<li>
<a href="profile.html">
<span>Profile</span>
</a>
</li>
<li>
<label for="darkmode">
<input type="checkbox" id="darkmode" class="darkmode">
<b>๐</b>
</label>
</li>
</ul>
</nav>
</body>
</html>
The code displayed above serves as an example. You can follow it as a guide.
Brief explanation: As you can see in the last line, the 'input' and 'b' elements are placed within the 'li' item. This is part of the dark/light mode feature, which I will explain in the final section.
Next, we add the 'main' element to make it resemble a real project. Hereโs the HTML code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>home</title>
<link rel="stylesheet" href="style.css">
<script type="text/javascript" src="app.js" defer></script>
</head>
<body>
<nav id="sidebar">
<ul>
<li>
<button id="toggle-btn" onclick=toggleSideBar() >close</button>
</li>
<li class="active">
<a href="index.html">
<span>Home</span>
</a>
</li>
<li>
<a href="dashboard.html">
<span>Dashboard</span>
</a>
</li>
<li>
<button onclick=toggleSubMenu(this) class="dropdown-btn">
<span>create</span>
</button>
<ul class="sub-menu">
<div>
<li>
<a href="">folder</a>
</li>
<li>
<a href="">document</a>
</li>
<li>
<a href="">project</a>
</li>
</div>
</ul>
</li>
<li>
<a href="calendar.html">
<span>Calendar</span>
</a>
</li>
<li>
<a href="profile.html">
<span>Profile</span>
</a>
</li>
<li>
<label for="darkmode">
<input type="checkbox" id="darkmode" class="darkmode">
<b>๐</b>
</label>
</li>
</ul>
</nav>
<main>
<div class="container">
<h2>Hello world</h2>
<p>Lorem, ipsum dolor sit amet consectetur adipisicing elit. Obcaecati quas totam voluptate sit at voluptatum culpa, laborum dignissimos nobis amet inventore perspiciatis, veniam consectetur, unde molestiae ratione odit! Accusantium, blanditiis!</p>
</div>
<div class="container">
<h2>example heading</h2>
<p>Lorem ipsum dolor, sit amet consectetur adipisicing elit. Mollitia facere saepe dolor nulla error commodi minus. Impedit saepe commodi voluptates in blanditiis, quo sit aperiam sunt deleniti praesentium repellat modi?</p>
</div>
<div class="container">
<h2>lorem ipsum</h2>
<p>Lorem ipsum dolor, sit amet consectetur adipisicing elit. Porro architecto excepturi provident ut possimus similique alias esse voluptatem inventore atque doloremque sed natus, vel quisquam est nesciunt soluta ea impedit.</p>
</div>
</main>
</body>
</html>
Then, we need to add styling to our project to make it look more polished. You can follow the code below.
:root {
--base-clr: #11121a;
--line-clr: #42434a;
--hover-clr: #222533;
--text-clr: #e6e6ef;
--accent-clr: #5e63ff;
--secondary-text-clr: #b0b3c1;
}
*{
margin: 0;
padding: 0;
}
html {
font-family: 'Courier New', Courier, monospace;
line-height: 1.5rem;
}
body{
min-height: 100vh;
min-height: 100dvh;
background-color: var(--base-clr);
color: var(--text-clr);
display: grid;
grid-template-columns: auto 1fr;
}
body:has(#darkmode:checked){
background-color: var(--text-clr);
color: var(--base-clr);
p {
color: var(--base-clr);
}
#sidebar {
background-color: var(--base-clr);
}
}
#sidebar {
box-sizing: border-box;
height: 100vh;
width: 250px;
padding: 5px 1em;
background-color: var(--base-clr);
border-right: 1px solid var(--line-clr);
position: sticky;
top: 0;
align-self: start;
transition: 300ms ease-in-out;
overflow: hidden;
box-shadow: 5px 5px 5px rgb(44, 42, 42);
}
#sidebar.close {
padding: 5px;
width: 60px;
span {
display: none;
}
a {
display: none;
}
.dropdown-btn {
display: none;
}
.darkmode {
display: none;
}
b {
display: none;
}
}
#sidebar a span, #sidebar .dropdown-btn span, #sidebar b {
flex-grow: 1;
}
#sidebar ul {
list-style: none;
}
.darkmode {
display: none;
}
#sidebar > ul > li:first-child{
display: flex;
justify-content: flex-end;
margin-bottom: 16px;
}
#sidebar ul li.active a{
color: var(--accent-clr);
}
#sidebar a, #sidebar .dropdown-btn, #sidebar b {
border-radius: .5em;
padding: .85em;
text-decoration: none;
color: var(--text-clr);
display: flex;
align-items: center;
gap: 1em;
}
#sidebar a:hover, #sidebar .dropdown-btn:hover{
background-color: var(--hover-clr);
}
#sidebar .sub-menu{
display: grid;
grid-template-rows: 0fr;
transition: 300ms ease-in-out;
> div{
overflow: hidden;
}
}
#sidebar .sub-menu a{
padding-left: 2em;
}
#sidebar .sub-menu.show {
grid-template-rows: 1fr;
}
#toggle-btn {
margin: 4px;
margin-left: auto;
padding: 8px;
border: none;
border-radius: .5em;
background: none;
cursor: pointer;
color: var(--text-clr);
}
#toggle-btn:hover {
background-color: var(--hover-clr);
}
.dropdown-btn{
width: 100%;
text-align: left;
background: none;
border: none;
font: inherit;
cursor: pointer;
font-weight: bolder;
}
main {
padding: min(30px,7%);
}
main p {
color: var(--secondary-text-clr);
margin-top: 5px;
margin-bottom: 15px;
}
.container{
border: 1px solid var(--line-clr);
border-radius: 1em;
margin-bottom: 20px;
padding: min(3em, 15%);
h2, p {margin-top: 1em;}
}
Based on the code above, it is not responsive yet. So, we will make it responsive. Hereโs the CSS code.
@media(max-width: 800px) {
body {
grid-template-columns: 1fr;
}
main{
padding: 2em 1em 60px 1em;
}
.container {
border: none;
padding: 0;
}
#sidebar{
height: 60px;
width: 100%;
border-right: none;
border-top: 1px solid var(--line-clr);
box-shadow: 10px 10px 10px 10px rgb(44, 42, 42);
position: fixed;
top: unset;
bottom: 0;
padding: 0;
>ul{
display: grid;
grid-auto-flow: column;
align-items: center;
list-style: none;
padding: 0;
margin: 0;
width: 100%;
gap: 10px;
}
ul li {
height: 100%;
display: inline-flex;
font-size: small;
}
ul a, ul .dropdown-btn, ul b{
width: 50px;
height: 60px;
padding: 0;
border-radius: 0;
justify-content: center;
}
.dropdown-btn {
margin-left: 5px;
}
.dropdown-btn:hover{
background-color: inherit;
transform: none;
color: var(--secondary-text-clr);
}
a:hover{
background-color: inherit;
transform: none;
color: var(--secondary-text-clr)
}
#toggle-btn, .sub-menu {
display: none;
}
ul li .sub-menu.show {
position: fixed;
box-sizing: border-box;
height: 60px;
width: 100%;
background-color: var(--hover-clr);
border-top: 1px solid var(--line-clr);
display: flex;
justify-content: center;
bottom: 60px;
left: 0;
>div{
overflow-x:auto ;
}
li{
display: inline-flex;
}
a{
box-sizing: border-box;
padding: 1em;
width: auto;
justify-content: center;
}
}
}
}
It's will look like this.
Next, we will add JavaScript logic to make it interactive. We will create a toggle button function to allow the sidebar to open and close. Additionally, we will implement a toggle function to change the icon from 'โ๏ธ' to '๐'. Hereโs the JavaScript code.
const toggleButton = document.getElementById('toggle-btn');
const sidebar = document.getElementById('sidebar');
const darkModeToggle = document.getElementById('darkmode');
const bElement = document.querySelector('b');
darkModeToggle.addEventListener('change', ()=> {
if (darkModeToggle.checked) {
bElement.textContent = '๐';
} else {
bElement.textContent = '๐';
}
});
function toggleSideBar() {
sidebar.classList.toggle('close')
if(sidebar.classList.contains('close')) {
toggleButton.textContent='open'
} else {
toggleButton.textContent='close'
}
}
const toggleSubMenu = (button) => {
button.nextElementSibling.classList.toggle('show')
}
Next, you can run your code from the terminal.
The result will look like this
So,How does the dark/light mode work?
As you can see, we have added an 'input' element inside the 'li' item. The 'input' element is a checkbox, and we used the :has() pseudo-class to apply specific styles to the body.
body:has(#darkmode:checked){
background-color: var(--text-clr);
color: var(--base-clr);
p {
color: var(--base-clr);
}
#sidebar {
background-color: var(--base-clr);
}
}
This means that when users click the checkbox, the background will change.
Thank you for reading! If you found this post helpful, please spread the word.
We'd love to hear from you! Let us know how we can better assist you by sharing your suggestions.
Contact us: https://muhamadalzika.com/about Email: zikafakk@gmail.com