How to create a simple calculator using HTML, CSS, JavaScript.
Posted on 2024-09-04
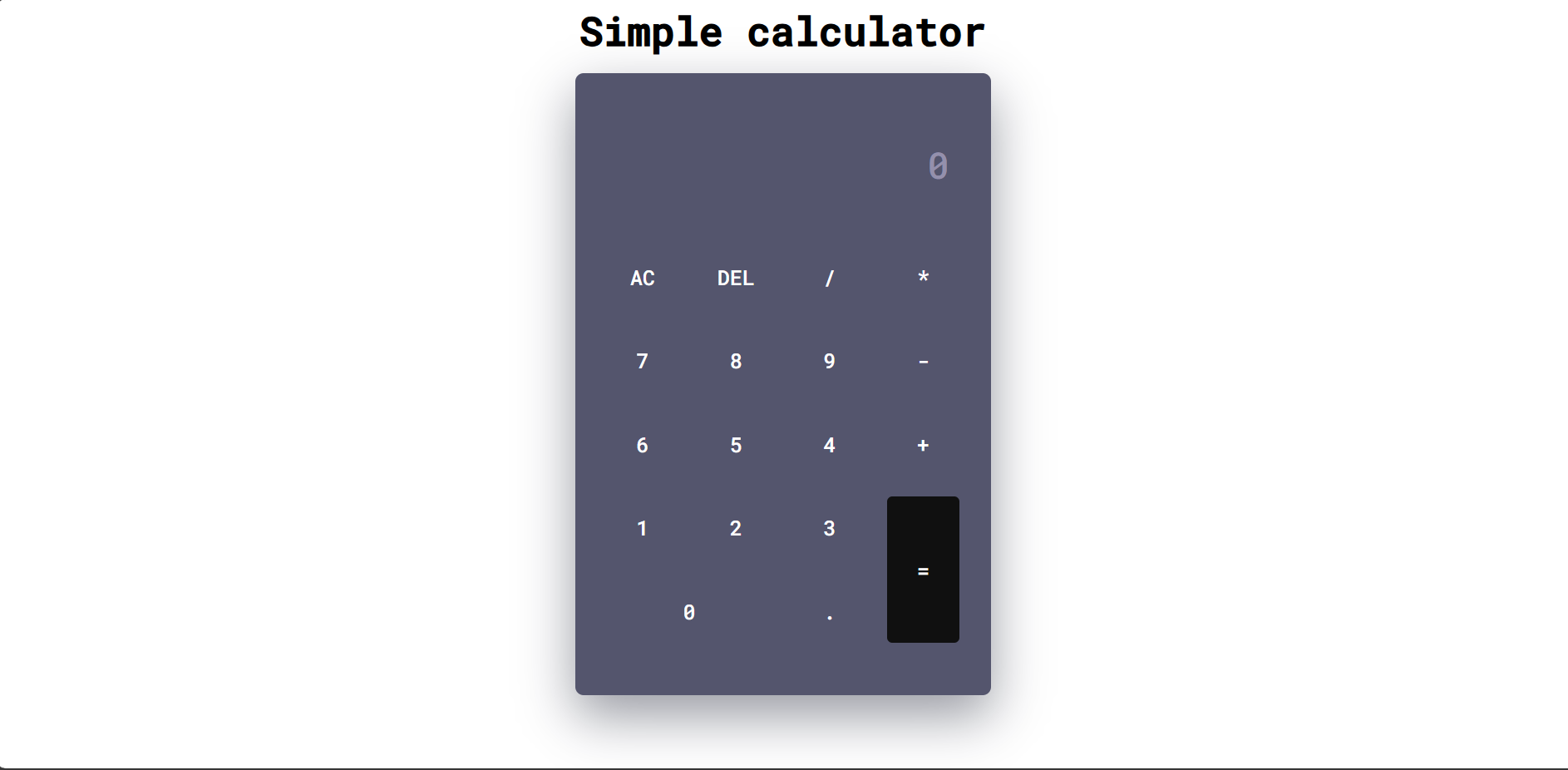
Hello guys👋, welcome back! In this tutorial, we are going to learn how to create a calculator using HTML, CSS, and JavaScript. The purpose of this tutorial is to improve our programming logic.
We’ll need three files in a folder. You can name the folder 'simple-calculator'. Inside, create three files: an HTML file, a CSS file, and a JavaScript file. First, we’ll write in the HTML file, which we’ll name 'index.html'. Next, we’ll create the CSS file and name it 'style.css'. Finally, we’ll create the JavaScript file and name it 'index.js'.
Let's start with the HTML code. Read also How to create a responsive navbar in Next.js.
<!DOCTYPE html>
<html lang="en">
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Calculator</title>
<!-- Google Font -->
<link
href="https://fonts.googleapis.com/css2?family=Roboto+Mono:wght@500&display=swap"
rel="stylesheet"
/>
<!-- Stylesheet -->
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div class="h1Class">
<h1>Simple calculator</h1>
</div>
<div class="calculator">
<div class="display">
<input type="text" placeholder="0" id="input" disabled />
</div>
<div class="buttons">
<!-- Full Erase -->
<input type="button" value="AC" id="clear" />
<!-- Erase Single Value -->
<input type="button" value="DEL" id="erase" />
<input type="button" value="/" class="input-button" />
<input type="button" value="*" class="input-button" />
<input type="button" value="7" class="input-button" />
<input type="button" value="8" class="input-button" />
<input type="button" value="9" class="input-button" />
<input type="button" value="-" class="input-button" />
<input type="button" value="6" class="input-button" />
<input type="button" value="5" class="input-button" />
<input type="button" value="4" class="input-button" />
<input type="button" value="+" class="input-button" />
<input type="button" value="1" class="input-button" />
<input type="button" value="2" class="input-button" />
<input type="button" value="3" class="input-button" />
<input type="button" value="=" id="equal" />
<input type="button" value="0" class="input-button" />
<input type="button" value="." class="input-button" />
</div>
</div>
<!-- Script -->
<script src="index.js"></script>
</body>
</html>
In the HTML code, within the
* {
padding: 0;
margin: 0;
box-sizing: border-box;
font-family: "Roboto Mono", monospace;
}
body {
height: 100vh;
}
.calculator {
width: 400px;
background-color: #54556d;
padding: 50px 30px;
position: absolute;
transform: translate(-50%, -50%);
top: 50%;
left: 50%;
border-radius: 8px;
box-shadow: 0 20px 50px rgba(0, 5, 24, 0.4);
}
.display {
width: 100%;
}
.display input {
width: 100%;
padding: 15px 10px;
text-align: right;
border: none;
background-color: transparent;
color: #ffffff;
font-size: 35px;
}
.h1Class {
text-align: center;
font-size: larger;
font-weight: bold;
font-family: monospace;
margin: 5px;
flex: auto;
justify-items: center;
}
.display input::placeholder {
color: #9490ac;
}
.buttons {
display: grid;
grid-template-columns: repeat(4, 1fr);
grid-gap: 20px;
margin-top: 40px;
}
.buttons input[type="button"] {
font-size: 20px;
padding: 17px;
border: none;
background-color: transparent;
color: #ffffff;
cursor: pointer;
border-radius: 5px;
}
.buttons input[type="button"]:hover {
box-shadow: 0 8px 25px #000d2e;
}
input[type="button"]#equal {
grid-row: span 2;
background-color: #101010;
}
input[type="button"][value="0"] {
grid-column: span 2;
}
Now, to add functionality to the calculator, we use JavaScript. We start by creating a variable called equal_pressed, which is initially set to zero. Every time the equal button is pressed, the value of equal_pressed is changed to one. This means that once an evaluation is completed and the equal button is pressed, the display will clear itself when the user enters a new number or operator for a new calculation. We also add a click event listener to the clear button (AC). When AC is pressed, the entire display is cleared. Similarly, we add a click event listener to the erase button. Here, we use the substr method to delete or remove the last entered digit from the display.
let equal_pressed = 0;
//Refer all buttons excluding AC and DEL
let button_input = document.querySelectorAll(".input-button");
//Refer input,equal,clear and erase
let input = document.getElementById("input");
let equal = document.getElementById("equal");
let clear = document.getElementById("clear");
let erase = document.getElementById("erase");
window.onload = () => {
input.value = "";
};
//Access each class using forEach
button_input.forEach((button_class) => {
button_class.addEventListener("click", () => {
if (equal_pressed == 1) {
input.value = "";
equal_pressed = 0;
}
//display value of each button
input.value += button_class.value;
});
});
//Solve the user's input when clicked on equal sign
equal.addEventListener("click", () => {
equal_pressed = 1;
let inp_val = input.value;
try {
//evaluate user's input
let solution = eval(inp_val);
//True for natural numbers
//false for decimals
if (Number.isInteger(solution)) {
input.value = solution;
} else {
input.value = solution.toFixed(2);
}
} catch (err) {
//If user has entered invalid input
alert("Invalid Input");
}
});
//Clear Whole Input
clear.addEventListener("click", () => {
input.value = "";
});
//Erase Single Digit
erase.addEventListener("click", () => {
input.value = input.value.substr(0, input.value.length - 1);
});
The calculator has been made! We have been finished created a calculator using HTML CSS JavaScript. If you found this post helpful, please spread the word. We'd love to hear from you! Let us know how we can better assist you by sharing your suggestions.
Contact us: https://muhamadalzika.com/about Email: zikafakk@gmail.com